What are you required to be able to complete for the purpose of creating an app that is capable of recognizing living objects using React? (r) (r)
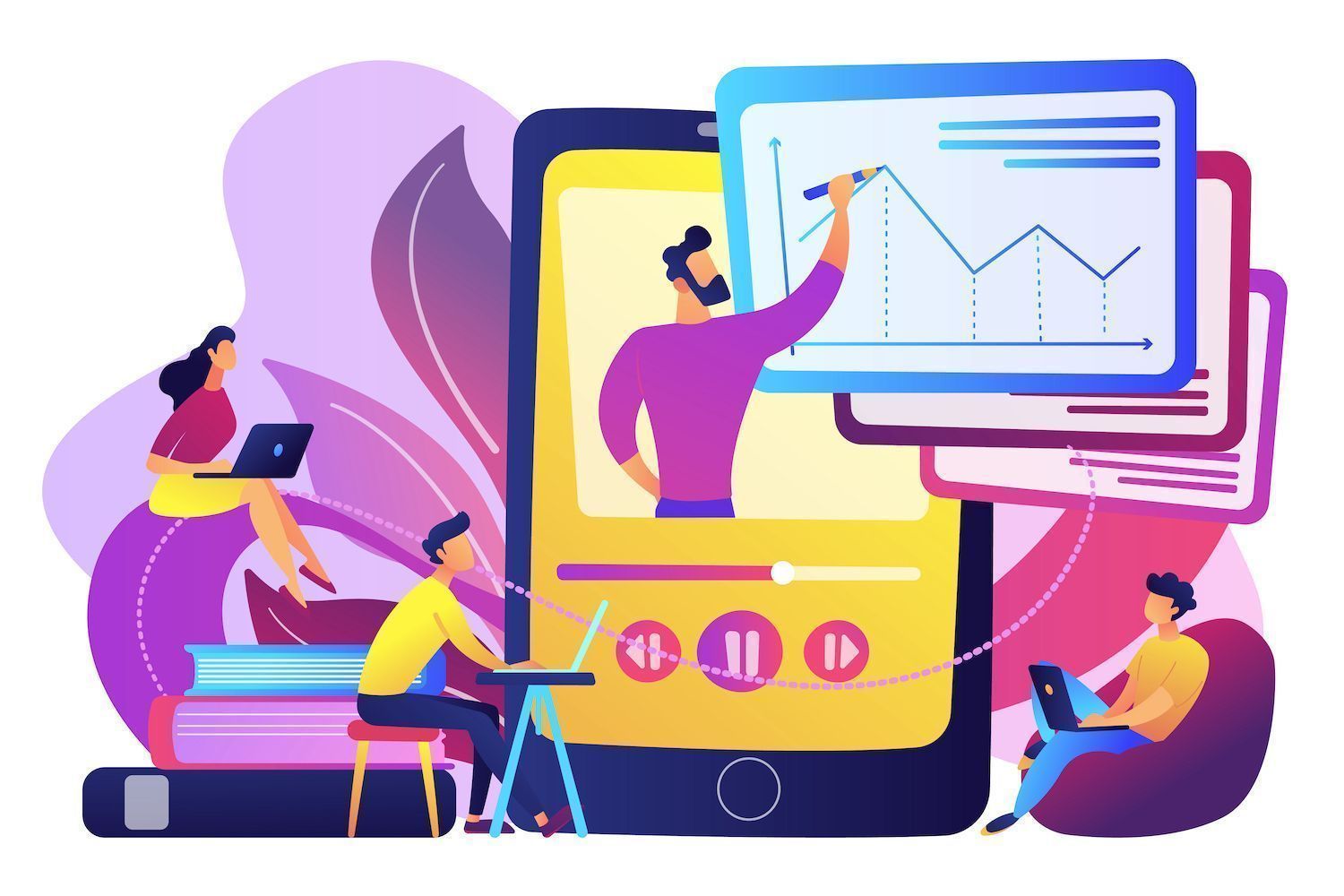
-sidebar-toc>
Cameras are becoming better and are becoming more sophisticated. The ability to identify objects in a flash is rapidly becoming a popular alternative. From self-driving cars to advanced equipment for surveillance to AR applications AR technology is used to diverse applications.
Computer Vision is the nebulous term used to describe a method that utilizes of cameras and computers for various purposes. This is, as mentioned previously it's a vast and intricate area. A lot of people aren't sure they're ready to participate actively in searching of things with a Google searching engine.
The scene
This is a comprehensive review of the top techniques that are used in this article:
- TensorFlow.js: TensorFlow.js is a JavaScript library that brings the benefits of machine-learning into your web browser. It allows you to download models which have been designed to accomplish the task of object recognition for the web browser. This eliminates the requirement to perform complex server-side processing.
- Coco SSD is an application that allows recognition of objects, which is taught. The application is also known by the title of Coco SSD which is a light model that is capable to detect the vast majority of objects that are often used. While Coco SSD is a powerful tool, you need to be aware that it was created by using various objects. If you're searching for the exact characteristics that you are able to identify and then create an individual model with TensorFlow.js using this article.
Rethink the design of a new React project
- Start a new React project. It's simple with these simple steps:
NPM create vite@latest -object detection React template
It will be the first React project that you can create by..
- Following that, you'll capable of downloading TensorFlow and Coco SSD. Coco SSD libraries will be downloaded using these commands are included in the project:
npm i @tensorflow-models/coco-ssd @tensorflow/tfjs
Now is the time to design your application.
Configuring the application
Before you write the code that will create the logic necessary for recognizing objects, examine the code that was written in this instruction. The user interface of the application might include:
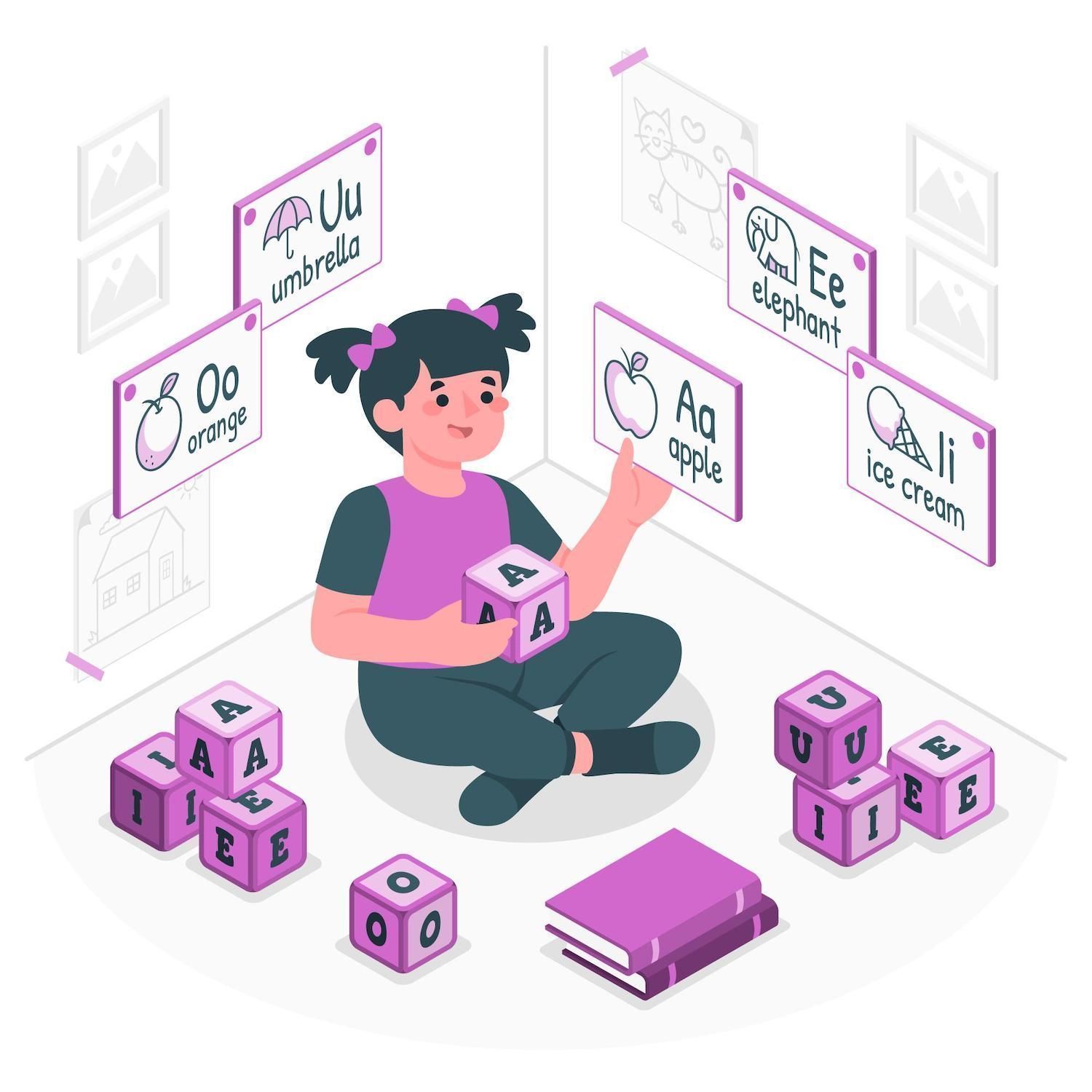
If users click the Start Webcam button upon clicking on the Start Webcam button, they're obliged to grant permission for the application to use webcam feeds. When permission is granted then the program will display the live stream from the webcam. The application can also detect any objects it sees in the stream. It forms an equilateral triangular form to show the things it observes within the feed. It is then able to label those things. It is then able identify the items.
First thing to complete is to create an user-friendly interface for your application. Then copy these steps into app.jsx. App.jsx file:
import ObjectDetection from './ObjectDetection'function App() return ( Image Object Detection ); Export default App
The code fragment functions as the page's header. The code also contains the custom component "ObjectDetection"
. It receives the feed from a camera and determine that the object is that are on the spur at exactly the right time.
To create this component, make an entirely new file that has the title ObjectDetection.jsx in your homedirectory and then copy the following code in it:
UseEffect useState and'react'. Const objectDetection = () Const videoRef = useRef(null) Const [isWebcamStartedsetIsWebcamStarted], useState(false) Const setWebcam to be in sync () = /StopWebcam () > // TODO; return ( isWebcamStarted? "Stop" : "Start" Webcam isWebcamStarted ? : ); ; export default ObjectDetection;
It is possible to implement the code for building the startWebcam. "startWebcam"
function:
const startWebcam = async () => try setIsWebcamStarted(true) const stream = await navigator.mediaDevices.getUserMedia( video: true ); if (videoRef.current) videoRef.current.srcObject = stream; catch (error) setIsWebcamStarted(false) console.error('Error accessing webcam:', error); ;
The system asks users to allow permission to access the camera. After granting access it modifies the stream of video from the webcam. video
will show the live stream of the webcam for anyone who is linked to the camera.
If the application isn't capable of connecting to feeds of the camera (possibly because there isn't of a webcam on the device, or another reason why the user wasn't granted access) it will display an error message in the console. The console could display an error message which will explain the source of this issue to the user.
The next step is replacing stopWebcam by stopWebcam. stopWebcam
functions using this code:
const stopWebcam = () => const video = videoRef.current; if (video) const stream = video.srcObject; const tracks = stream.getTracks(); tracks.forEach((track) => track.stop(); ); video.srcObject = null; setPredictions([]) setIsWebcamStarted(false) ;
The code is able to scan for videos which can be accessed via webcam objects. webcam
object. It will stop every one of them. After that, it'll modify the camera's
position to its actual number.
.
Similar to the scenario. It's possible to start the program and verify that it's able to connect to and show the feed from the webcam.
The code should be put into your index.css file to ensure that the application appears exactly as the one you saw earlier.
#root font-family: Inter, system-ui, Avenir, Helvetica, Arial, sans-serif; line-height: 1.5; font-weight: 400; color-scheme: light dark; color: rgba(255, 255, 255, 0.87); background-color: #242424; min-width: 100vw; min-height: 100vh; font-synthesis: none; text-rendering: optimizeLegibility; -webkit-font-smoothing: antialiased; -moz-osx-font-smoothing: grayscale; a font-weight: 500; color: #646cff; text-decoration: inherit; a:hover color: #535bf2; body margin: 0; display: flex; place-items: center; min-width: 100vw; min-height: 100vh; h1 font-size: 3.2em; line-height: 1.1; button border-radius: 8px; border: 1px solid transparent; padding: 0.6em 1.2em; font-size: 1em; font-weight: 500; font-family: inherit; background-color: #1a1a1a; cursor: pointer; transition: border-color 0.25s; button:hover border-color: #646cff; button:focus, button:focus-visible outline: 4px auto -webkit-focus-ring-color; @media (prefers-color-scheme: light) :root color: #213547; background-color: #ffffff; a:hover color: #747bff; button background-color: #f9f9f9; .app width: 100%; display: flex; justify-content: center; align-items: center; flex-direction: column; .object-detection width: 100%; display: flex; flex-direction: column; align-items: center; justify-content: center; .buttons width: 100%; display: flex; justify-content: center; align-items: center; flex-direction: row; button margin: 2px; div margin: 4px;
The app.css file should be eliminated. App.css file to ensure you do not damage your components' appearance. Now you are ready to integrate the necessary technology for real-time object recognition to your application.
Implement real-time detection for objects
- The first step is to load the data into Tensorflow together with Coco SSD in the middle of ObjectDetection.jsx :
import * as cocoSsd from '@tensorflow-models/coco-ssd'; import '@tensorflow/tfjs';
- Create a new condition for add a new condition to the
the ObjectDetection
component in order to keep the prediction array created by the Coco SSD model. Coco SSD model: Coco SSD model:
const [predictions setPredictions, useStatesetPredictions, useState ([]);
- Once you have that done, you will be competent to develop an application that is downloaded into the Coco SSD model. Coco SSD model. It is loaded onto Coco SSD model, loads onto Coco SSD model, collects footage, and then predicts:
const predictObject = async () => const model = await cocoSsd.load(); model.detect(videoRef.current).then((predictions) => setPredictions(predictions); ) .catch(err => console.error(err) ); ;
The software makes use of footage taken from the video feed to create forecasts of things that are in the feed. It provides users with a wide range of items that are likely to be seen. Every object is labeled which includes a percentage the degree of certainty, along with numbers which indicate the location of the object within the video's frame.
It is crucial to enable this feature to run videos according to the sequence in which frames are added. The forecasts will be later used and saved in this forecasts
condition. Forecasts will display boxes with labels for every identified object within the live video stream. live-stream view.
- In this case, you'll be able use
the settingInterval
function to enable this function at a regular interval. Also, you must be certain that the feature is not activated when the user is shut off updates on their camera. To avoid this, you must use to use theClearInterval
feature of JavaScript.Add your state container along with hooks for useEffect into useEffecteffect
hooks inside theelement, the detector of the objects
element, in order to build anpredict
function. The program runs indefinitely while the camera is in use. However, it will be taken off the camera when it is switched off.
const [detectionInterval, setDetectionInterval] = useState() useEffect(() => if (isWebcamStarted) setDetectionInterval(setInterval(predictObject, 500)) else if (detectionInterval) clearInterval(detectionInterval) setDetectionInterval(null) , [isWebcamStarted])
This application was designed to detect items in images taken by the camera every 500 milliseconds. You can alter the number of milliseconds per second, based on how you'd like to speed up detection of objects, but you should take into consideration the consequences of using often. It may result in the program consuming large amounts of memory in the browser.
- When you've got the data to make your forecast, you'll utilize the data you've collected to estimate the forecast. If you've estimated some aspects that you forecasted, these can be used to label containers. Forecasts are a great way to highlight your label in containers and to show the label in the live feed within the film. To do this, you must change your
return
declaration in order to enable yourlabel detection.
Enter the following details:
Return ( Is WebcamStarted ? "Stop" : "Start" Webcam isWebcamStarted ? : /* Add the tags below to show a label using the p element and a box using the div element */ predictions.length > 0 && ( predictions.map(prediction => return prediction.class + ' - with ' + Math.round(parseFloat(prediction.score) * 100) + '% confidence. ' > ) ) /* Add the tags below to show a list of predictions to user */ predictions.length > 0 && ( Predictions: predictions.map((prediction, index) => ( `$prediction.class ($(prediction.score * 100).toFixed(2)%)` )) ) );
The program will display a list of forecasts underneath the feeds from the webcam. The program makes an empty region surrounding the object that is forecasted based on the coordinates provided by Coco SSD as well as names on the bottom of every box.
- for styling the labels and boxes to properly style labels and boxes for styling the labels and boxes correctly, you must include this code into the index.css Index.css index.css document. index.css file:
.feed position: relative; p position: absolute; padding: 5px; background-color: rgba(255, 111, 0, 0.85); color: #FFF; border: 1px dashed rgba(255, 255, 255, 0.7); z-index: 2; font-size: 12px; margin: 0; .marker background: rgba(0, 255, 0, 0.25); border: 1px dashed #fff; z-index: 1; position: absolute;
The application is completed. It's finished. The program is functioning properly. this server since it was built to test the functionality of the program. What happens after it has been completed
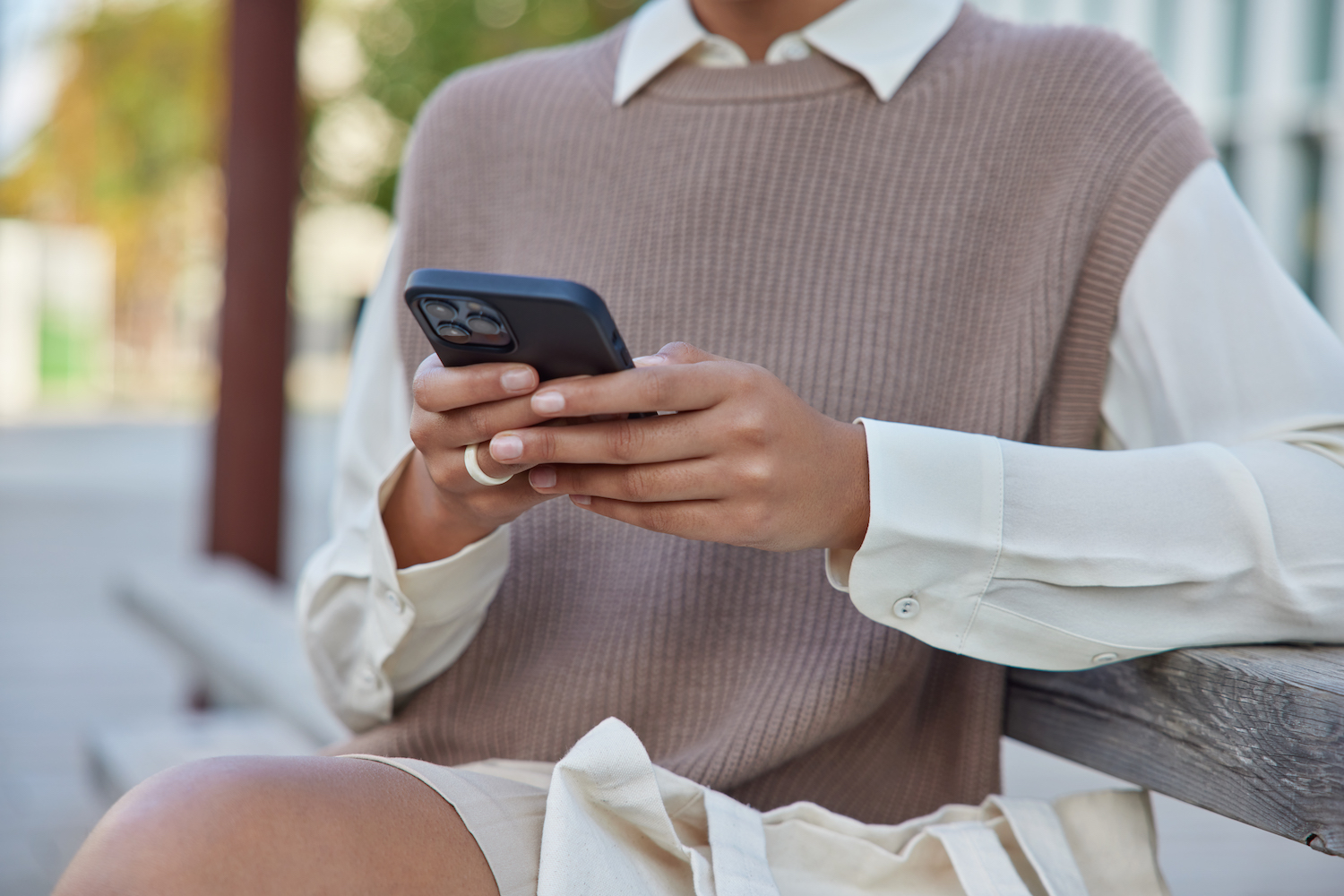
The complete program is accessible on this repository GitHub. GitHub repository.
Install the app
If your repository in Git is functioning and operational you can take these steps to set up Git :
- Create or register an account in order that you can login to Your Dashboard. My dashboard.
- It is necessary to authorize Git service providers.
- Select those static websites from the sidebar left. Choose Add Website. Choose to include the site.
- Choose which branch you would like to join and then the repository you want to access.
- Assign a unique name to your site.
- Set up the building's settings as per the following design:
- Command to build:
yarn build
orNPM build
- Node version:
20.2.0
- Publish directory:
dist
- Then, click Create site.
When the application is developed after which the app is launched, it's possible to click "Visit the website" from the dashboard to open the application. It is possible to test the app using multiple cameras across different platforms to test the capabilities of the application.
Summary
It has had a huge accomplishment in the development of machine-learning technology to recognize objects that works in real time along with live-time software that was created with React, TensorFlow.js, and . It allows you to explore the potential for computer-generated vision. It also lets you make interactive experiences with the web browser.
Take note you are using this model. Coco SSD model we used as a base. If you're interested in exploring the various options, it is worth exploring the possibility of being in an position to change the method of identifying objects by using TensorFlow.js that allows you to alter how the program detects objects that best satisfy the particular requirements of the company that you are employed by.
There's no limit to the possibilities you have to develop! This app could be the base for creating new and innovative apps like Augmented Reality Experiences and also advanced surveillance tools. If you're in the event of launching your application using a safe platform, you'll be in a position to make your app open to all users around the globe and watch the capabilities of computer vision emerge into the spotlight.
What's the toughest problem you've had confront and you think that the real-time detection of objects might be able to help solve? Share your experiences in the comments section in the comments section below!
Kumar Harsh
Kumar is the author of technical software. He owns and runs his house in India. He's an expert in JavaScript as well as DevOps. Learn more regarding the topic on his web site.
The original article was published on this website.
The story was published on this website.
The post was made by the writer on the blog.
The post was published on this blog.
The article was first spotted here. this website
Article was posted on this website
This post was first seen on here