Mastering React Conditional Rendering Deep Dive (r)
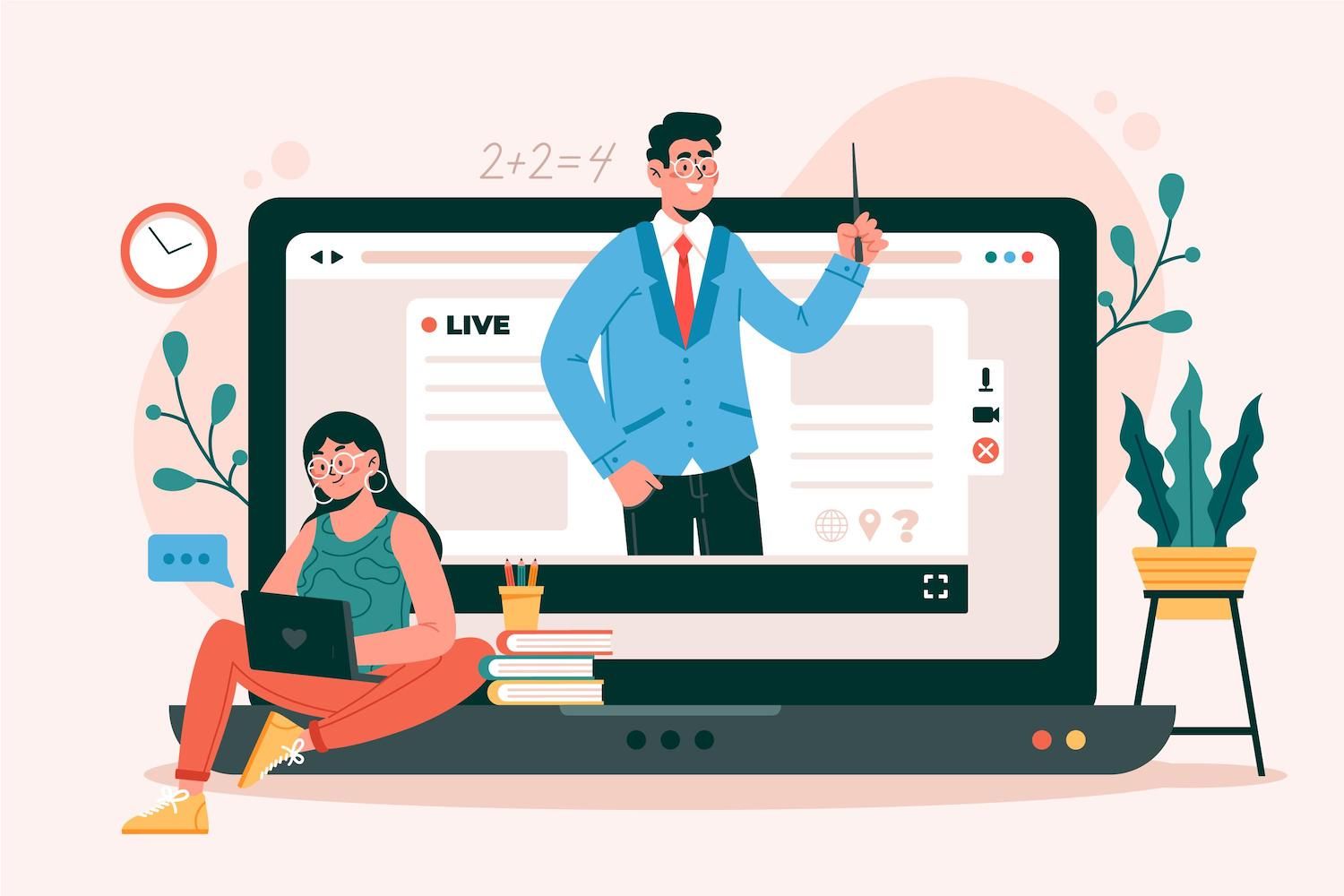
Send this to
Conditional rendering could be described as a vital feature of React which allows developers to render elements in response to certain conditions.
It's an essential notion that is crucial in building websites that are both dynamic and captivating.
In this complete guide We are going to dive deep into the world of conditional rendering using React. It will discuss the basics as well as advanced techniques and will provide examples to assist in comprehending.
React's perception of Conditional Rendering
It's extremely useful for situations in which you want to deactivate or block certain UI elements, alter the appearance of your website or present diverse variants of the page in response to the interaction with users.
Conditional rendering is crucial for React applications since it allows the creation of dynamic and interactive user interfaces that react to any modifications in the data or interaction, in real time.
The Fundamentals of Conditional rendering
There are a variety of basic techniques you can use to make conditional rendering using React. In this article, we will go over every one of them thoroughly.
By using the if expression to achieve the conditional rendering
One of the simplest ways to use the conditional rendering feature within React is to make use of the traditional "when"
statement.
When (condition) Return Expression 1. If not, return Expression 2.
The the If
clause is an excellent choice in the rendering()
method to ensure that your content is displayed in a particular manner that is in line with the specifics of the underpinning.
For an illustration what you are trying to illustrate, use the if statement to show the spinning wheel while you wait for information to be loaded:
import useState, useEffect from 'react'; import Spinner from './Spinner'; const MyComponent = () => const [isLoading, setIsLoading] = useState(true); const [data, setData] = useState(null); useEffect(() => // Fetch data from an API fetch('https://example.com/data') .then((response) => response.json()) .then((data) => setData(data); setIsLoading(false); ); , []); if (isLoading) return ; return /* Render the data here */; ; export default MyComponent;
In this scenario, MyComponent
fetches information from an API by making use of. utilizeEffect
hook. As you wait for the information to be loaded, you can use the Spinner component is controlled by an If
clause.
A different example is rendering to serve as a fallback option in the event that rendering is not successful due to an error. your component.
const MyComponent const MyComponent ( data ) * export default MyComponent
In this case, it's possible to use MyComponent. MyComponent
is a component that accepts the prop for information
prop. If the prop is not valid and it will display an error message.
prop isn't valid it will show an error message along with an "If"
statement.
Furthermore, you may display different content to multiple users roles through your whenever
clause:
const MyComponent = ( user ) * Export default MyComponent;
It's feasible to construct the MyComponent
which makes use of a human for the
device. Based on the user.role
property, the contents are displayed employing an "If"
clause.
Utilizing the Ternary Operator to perform Conditional rendering
The most efficient method for making use of conditional rendering inside React is using the operator ternary (?) inside JSX.
The ternary operator allows users to construct an Inline If-Else with three operands. The first operand is known as the condition. The third and second operands make up the expressions. When the condition is valid,
then the first expression is executed. If there is no requirement, the second one is executed.
You can, for instance, render a frame of a prop
import ComponentA from './ComponentA'; import ComponentB from './ComponentB'; const ExampleComponent = ( shouldRenderComponentA ) => return ( shouldRenderComponentA ? : ); ; export default ExampleComponent;
In this code, we have an ExampleComponent
that takes a prop called shouldRenderComponentA
. This operator is utilized to perform the rendering ComponentA
or ComponentB
depending on the prop's value.
It is possible to create other texts based on:
import useState from 'react'; const ExampleComponent = () => const [showMessage, setShowMessage] = useState(false); return ( setShowMessage(!showMessage)> showMessage ? show message" message" message" Hello, world! : null ); ; export default ExampleComponent;
In this instance, it's the ternary operator which renders the text in a different way depending on showMessage's showMessage
status. When the button is clicked then the value of showMessage showMessage
may be altered and text may be shown or hidden depending on the situation.
You can also create the loading spinner when you download data:
import useState, useEffect from 'react'; import Spinner from './Spinner'; const ExampleComponent = () => const [isLoading, setIsLoading] = useState(true); const [data, setData] = useState(null); useEffect(() => const fetchData = async () => const response = await fetch('https://jsonplaceholder.typicode.com/todos/1'); const jsonData = await response.json(); setData(jsonData); setIsLoading(false); ; fetchData(); , []); return ( isLoading ? : data.title ); ; export default ExampleComponent;
In this example it is employed to make use of the ternary operator rendering a spinning spinner as the data is being downloaded from an API. After the data has been download and rendered we then render the title of the property
property by using the operators of the ternary.
Utilizing Logical OR Operators to perform Conditional rendering
Additionally, it is possible to use also the AND ( &&
) in addition to OR ( ||
) operators for the rendering of conditional elements within React.
The logic AND operator allows the rendering of a component only if one of the conditions is met, while the logic OR operator allows rendering of an element when either or both of the requirements is met.
These are helpful in situations where you need to establish simple rules to determine what elements should be rendered or not. For instance when you have rendering a button, if the format is acceptable the format is acceptable, you could apply the logic AND operator to render it as follows:
import useState from 'react'; const FormComponent = () => const [formValues, setFormValues] = useState( username: "", password: "" ); const isFormValid = formValues.username && formValues.password; const handleSubmit = (event) => event.preventDefault(); // Submit form data ; return ( setFormValues( ...formValues, username: e.target.value ) /> setFormValues( ...formValues, password: e.target.value ) /> isFormValid && Submit ); ; export default FormComponent;
This is an example of FormComponent
with a form that has two input fields which allow users to input the details of a username
as well as password
. This form uses useState as the useState
hook that handles the value of the form and also it's isFormValid
variable that determines how much each field is in the form with value. By using the logic AND operator, (&&), it will render the button to submit only when the variable's value
is the case. This will ensure that the button is only able to be enabled if the confirmation of forms is successfully completed.
Like that, you can also use an OR operator to display an error message when the data load is not complete or display a message about an error when you encounter an error
import React, useEffect, useState from 'react'; Const DataComponent = () => const [data setData, data] = useState(null) Const [isLoading isLoading, setIsLoadingisLoading, setIsLoading] = useState(true) setErrorMessage, const, setErrorMessage] = the useState (''); UseEffect(() to fetch data, return to retrieve the data, []); return ( >ErrorMessage ? ( errorMessage ) : ( data.map((item) => ( item.name )) ) > ); ; export default DataComponent;
In this instance, DataComponent. DataComponent
is able to collect data using an API using fetch. The data is presented in an array. It uses an UtilizeState
hook to manage the state of the data, including its load status and error message. Utilizing the logic OR operator, (||), we are capable of displaying the error or loading message when one of the circumstances is satisfied. A message is displayed for the user to show what's happening while obtaining information.
Using this logic AND as well as OR operators to create the conditional content, React is reliable and a simple approach to deal with basic scenarios. It is however recommended to employ other strategies for tackling complex situations like changing
declarations in order to create more complexity in thinking.
Advanced Methods of Conditional Rendering
Conditional rendering within React is more difficult depending on the specifics of the software. They are sophisticated methods which can be used to render conditionally in more complex circumstances.
Making use of Switch Statements to render conditional content
While ternary, statements as well as operators are the most common methods to render conditional statements using the switching
phrase, they could work for situations involving multiple situations.
Here's an illustration
import React from'react";const MyComponent = (userType) =switch (userType) userType) "admin": Return Welcome admin user! The case 'user' returned"welcome to regular users!" The default is to return. Log in to find out more. ; ; export default MyComponent;
It can be seen that it is the switch
statement that will create the content according to the userType
prop's conditional. This method can prove useful in a myriad of situations and offers a more structured and understandable way to manage complicated algorithmic procedures.
Conditional rendering using React Router
React Router is described as an application well-known to the general population, which manages routing for clients who use React applications. React Router lets you make the component render in accordance to the conditions of your current route.
Here's a simple example of the implementation of the conditional rendering feature using React Router:
import useState from 'react'; import BrowserRouter as Router, Route, Switch from 'react-router-dom'; import Home from './components/Home'; import Login from './components/Login'; import Dashboard from './components/Dashboard'; import NotFound from './components/NotFound'; const App = () => const [isLoggedIn, setIsLoggedIn] = useState(false); return ( isLoggedIn ? ( ) : ( ) ); ; export default App;
In the code below in the case above it'll use it's logged-in
status to display a component if it's located in the Dashboard
component. However, it will do this only when the user is logged in and not it is the not-found
component when users have not signed in to their account. Login component Login
component will change its status to show that it's in a
state to alter to the state
when users log into their account.
The child prop in the component will be passed to Login via a Login
component as well as employing Login's settingIsLoggedIn
function. It is possible to add the Login component to use props without declaring it in the Login
component without specifying the whereabouts for the
prop.
Summary
Conditional rendering is a reliable method in React which allows you to alter the user interface based on different situations.
When you're considering how sophisticated the application's UI algorithm is, you're in the position to choose the algorithm best suited to your requirements.
Be sure to keep your code tidy, neat and clean, as well as ensuring that you examine thoroughly your algorithm for conditional rendering to make sure it is functioning exactly the way you like it to do in various circumstances.
The post was published on this site.
The post first appeared here. this website
Article was posted on here