How do you build an app that detects live objects using React? (r) (r)
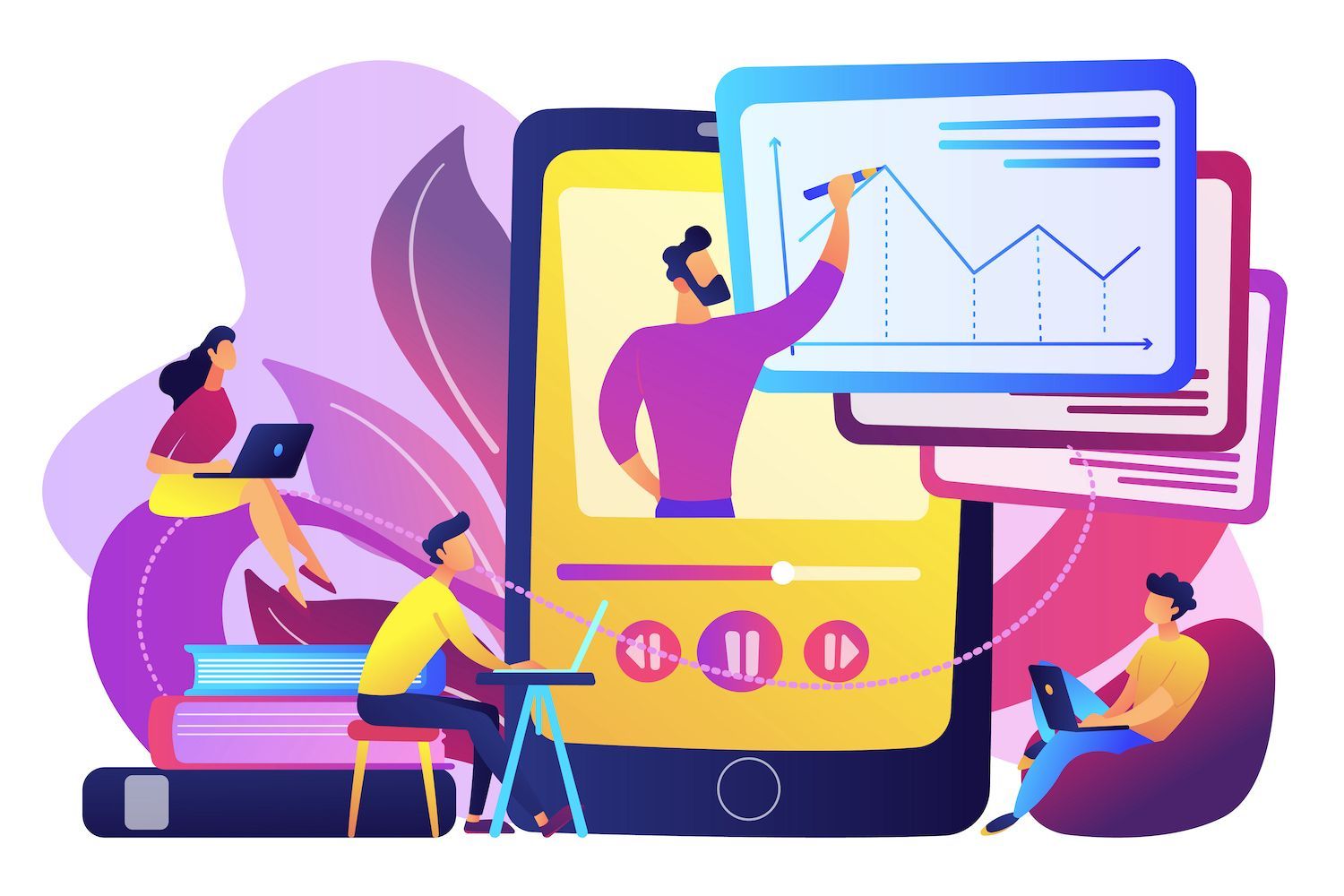
-sidebar-toc>
Since cameras have improved and advanced, real-time object detection is becoming a well-known option. From autonomous cars to smart surveillance systems to augmented reality applications, this technology could be employed in a myriad of scenarios.
Computer vision is an obscure name for the technique which makes use of cameras and computers in carrying out operations like the ones previously mentioned, is an enormous and intricate subject. You may not realize that it is feasible to start getting involved in the instantaneous detection of objects using your web browser.
The conditions
Below is a list of principal technologies utilized in this article:
- TensorFlow.js: TensorFlow.js is a JavaScript library that delivers the power of machine learning to the browser. It lets you load already-trained models which have been trained to perform object recognition and then run them in the browser. It eliminates the need to perform complex server-side processing.
- Coco SSD This application makes use of an object recognition model that has been trained known as Coco SSD, a lightweight model capable of recognising the vast majority of daily objects in real time. While Coco SSD is a powerful tool, you should be aware that it's trained using an entire set of objects. If you've got specific detection needs, you can create a customized model with TensorFlow.js using going through this instructional.
Start a new React project
- Make a brand new React project. You can do this with the help of this commands:
NPM create vite@latest -object detection React template
This will generate a base React project you are able to build by using vite.
- After that, then you can install TensorFlow along with The Coco SSD libraries by running these commands in the project:
npm i @tensorflow-models/coco-ssd @tensorflow/tfjs
It's time to start developing your app.
Configuring the app
Before writing the code to implement the logic for object detection, let's look at the logic created by this guide. The interface of this app may be as follows:
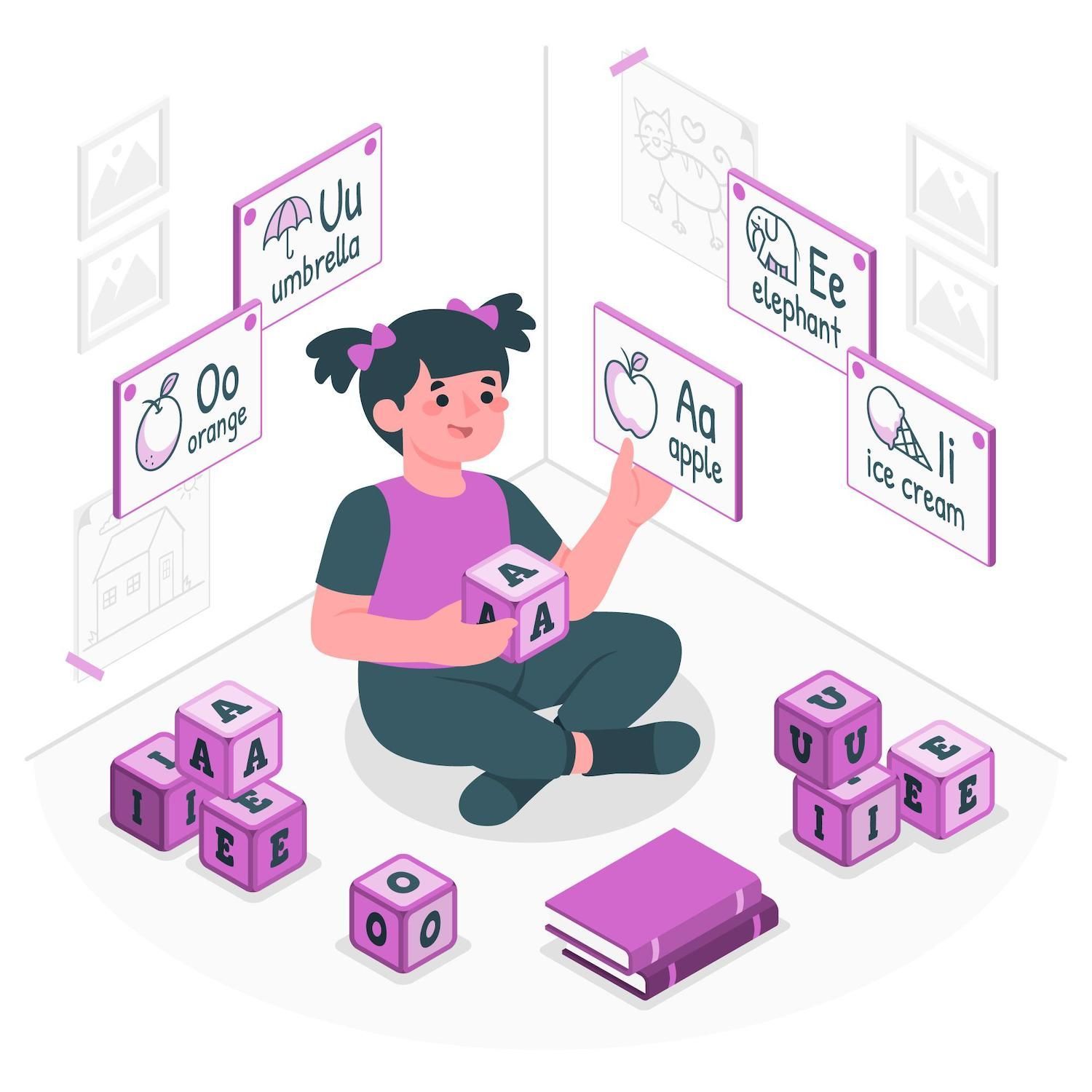
When users press the Start Webcam button, they're asked to allow the app to use the feed of webcams. When permission is granted, the app begins display the live feed of the webcam and recognizes the items it sees in the feed. After that, it creates an equilateral triangle to show the item it finds in the feed live and label them, and then.
For the first step, design an interface for users of your app by copying these instructions to app.jsx. App.jsx file:
import ObjectDetection from './ObjectDetection'function App() return ( Image Object Detection ); Export default App
This fragment of code defines the head of the page and also imports a custom component dubbed the ObjectDetection
. This component is responsible for taking a webcam's feed, and finding objects in real time.
In order to create this component create a brand-new document called ObjectDetection.jsx in your homedirectory and then copy the following code into it:
UseEffect, useRef, and useState from 'react'; Const objectDetection = () // const videoRef = useRef(null); const [isWebcamStarted ], setIsWebcamStarted] = useState(false) Const setWebcam to async () = // TODO ; const stopWebcam = () => // TODO ; return ( isWebcamStarted? "Stop" : "Start" Webcam isWebcamStarted ? : ); ; export default ObjectDetection;
Here's the code to implement startWebcam. "startWebcam"
function:
const startWebcam = async () => try setIsWebcamStarted(true) const stream = await navigator.mediaDevices.getUserMedia( video: true ); if (videoRef.current) videoRef.current.srcObject = stream; catch (error) setIsWebcamStarted(false) console.error('Error accessing webcam:', error); ;
The system will request users to allow an access right to their web camera and when granted permission it will change up the video
to show a live webcam feed to the user.
If the code fails accessing the webcam feed (possibly due to a lack of webcam on the current device or the reason that a user is not allowed access) it will print an error message to the console. You can use an error message that explains causes of failure to the user.
Next to replace this stopWebcam
function using the following code:
const stopWebcam = () => const video = videoRef.current; if (video) const stream = video.srcObject; const tracks = stream.getTracks(); tracks.forEach((track) => track.stop(); ); video.srcObject = null; setPredictions([]) setIsWebcamStarted(false) ;
The code scans for video streams accessible through the video
object and stops every one of the streams. Finally, it sets the isWebcamStarted
status to True
.
In this case, you can try running the app to check if you can access and view the feed from the webcam.
You must paste this code inside your index.css file to ensure that your application looks exactly like the one you have seen earlier:
#root font-family: Inter, system-ui, Avenir, Helvetica, Arial, sans-serif; line-height: 1.5; font-weight: 400; color-scheme: light dark; color: rgba(255, 255, 255, 0.87); background-color: #242424; min-width: 100vw; min-height: 100vh; font-synthesis: none; text-rendering: optimizeLegibility; -webkit-font-smoothing: antialiased; -moz-osx-font-smoothing: grayscale; a font-weight: 500; color: #646cff; text-decoration: inherit; a:hover color: #535bf2; body margin: 0; display: flex; place-items: center; min-width: 100vw; min-height: 100vh; h1 font-size: 3.2em; line-height: 1.1; button border-radius: 8px; border: 1px solid transparent; padding: 0.6em 1.2em; font-size: 1em; font-weight: 500; font-family: inherit; background-color: #1a1a1a; cursor: pointer; transition: border-color 0.25s; button:hover border-color: #646cff; button:focus, button:focus-visible outline: 4px auto -webkit-focus-ring-color; @media (prefers-color-scheme: light) :root color: #213547; background-color: #ffffff; a:hover color: #747bff; button background-color: #f9f9f9; .app width: 100%; display: flex; justify-content: center; align-items: center; flex-direction: column; .object-detection width: 100%; display: flex; flex-direction: column; align-items: center; justify-content: center; .buttons width: 100%; display: flex; justify-content: center; align-items: center; flex-direction: row; button margin: 2px; div margin: 4px;
Remove the App.css file to ensure that you don't mess up the style of your elements. Now you are ready to implement the necessary logic real-time object recognition in your application.
Set up real-time object detection
- The initial step is to incorporate the imports of Tensorflow and Coco SSD to the top of ObjectDetection.jsx :
import * as cocoSsd from '@tensorflow-models/coco-ssd'; import '@tensorflow/tfjs';
- Create a new condition in the
ObjectDetection
component, to save the prediction array generated by the Coco SSD model:
const [predictions setPredictions, useStatesetPredictions, useState ([]);
- You can then make a program to load in Coco SSD model. It then loads into Coco SSD model, collects the feed of video and then makes a prediction:
const predictObject = async () => const model = await cocoSsd.load(); model.detect(videoRef.current).then((predictions) => setPredictions(predictions); ) .catch(err => console.error(err) ); ;
This program uses the feed of video to make the predictions for objects in the feed. The program will give you an assortment of objects that are predicted, each containing a label that includes a % of confidence and a listing of numbers which indicate the position of the object within the frame of video.
It is essential to invoke this function to process video frames as they come and utilize the forecasts saved in the predictions
state to show boxes and labels for every identified object on the live stream of video.
- Then, you can use the
the setInterval
function that calls this function on a regular basis. You must also stop this function from being activated once the user has shut off the webcam feed. To prevent this from happening, use yourClearInterval
functionality of JavaScript.Add the container for state along with useEffect hooks to theeffect
hooks in theelement ObjectDetection
element to establish thepredictObject
Function that runs continuously whenever the webcam is activated and taken off the sitecam once it's turned off:
const [detectionInterval, setDetectionInterval] = useState() useEffect(() => if (isWebcamStarted) setDetectionInterval(setInterval(predictObject, 500)) else if (detectionInterval) clearInterval(detectionInterval) setDetectionInterval(null) , [isWebcamStarted])
This sets up the app to detect the objects present prior to the camera's view every 500 milliseconds. You can consider changing the number of milliseconds depending on the speed you'd like for the object detection speed to occur, but be aware of the possibility that using this too frequently can result in your application using a lot of memory in the browser.
- After you've collected the data for your prediction, you can use it to make
predictions. Once you have a prediction
state containers. You are able to use it to show the label and also it as a box in the live video feed. For this to happen it is necessary to change yourreturn
declaration of theobject detection
Provide the following details:
return ( isWebcamStarted ? "Stop" : "Start" Webcam isWebcamStarted ? : /* Add the tags below to show a label using the p element and a box using the div element */ predictions.length > 0 && ( predictions.map(prediction => return prediction.class + ' - with ' + Math.round(parseFloat(prediction.score) * 100) + '% confidence. ' > ) ) /* Add the tags below to show a list of predictions to user */ predictions.length > 0 && ( Predictions: predictions.map((prediction, index) => ( `$prediction.class ($(prediction.score * 100).toFixed(2)%)` )) ) );
The program displays the predictions list beneath the feed from the webcam. It will then build a box surrounding the predicted object, with the coordinates of Coco SSD as well as a name above the boxes.
- To style the boxes and label correctly, insert this code into index.css file: index.css file:
.feed position: relative; p position: absolute; padding: 5px; background-color: rgba(255, 111, 0, 0.85); color: #FFF; border: 1px dashed rgba(255, 255, 255, 0.7); z-index: 2; font-size: 12px; margin: 0; .marker background: rgba(0, 255, 0, 0.25); border: 1px dashed #fff; z-index: 1; position: absolute;
The application is complete. the application. It is now possible to restart the development server to test the software. What it will be like after the application is completed
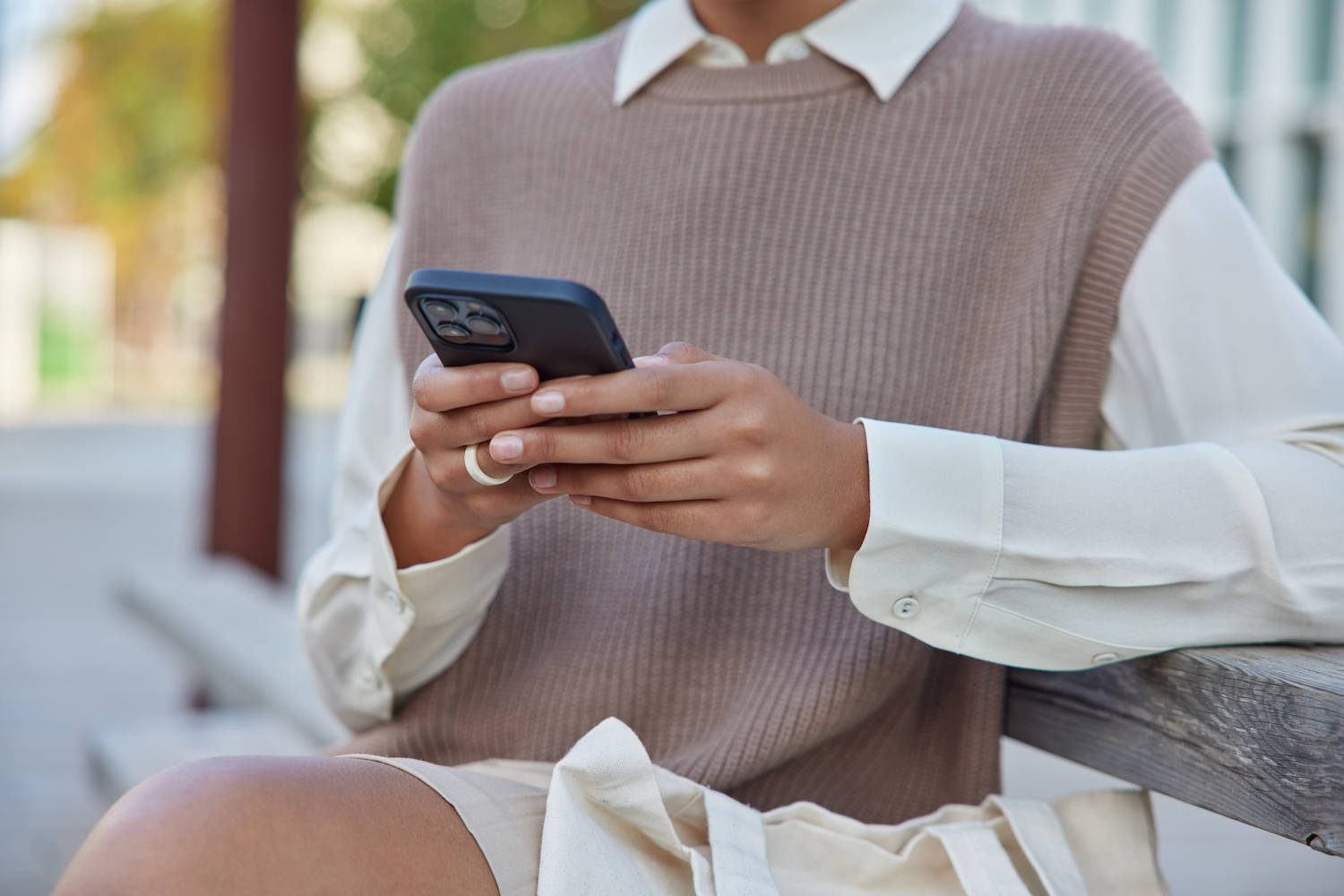
Complete code is available is available in the repository on GitHub. GitHub repository.
Deploy the completed app to
Once your repository on Git is operational then follow these steps to install the app on :
- Sign in or create an account to view the dashboard for Your dashboard. My dashboard.
- Authorize with your Git provider.
- Click on the static websites on the sidebar to the left, and then choose Add Site. Select Add Site.
- Choose the repository and branch that you want to be able to access from.
- Assign a unique name to your site.
- Include the build settings according to the following format:
- Build command:
yarn build
orNPM run build
- Node version:
20.2.0
- Publish directory:
dist
- Then, click Create site.
After the app has been deployed, you can select "Visit Website" from the dashboard in order to open the app. You can test the app on different devices that have cameras to determine what happens.
Summary
It's been successful to develop a live-time, object detection application using React, TensorFlow.js, and . It lets you explore the possibilities that lie in computer vision and develop interactive experiences in the user's web browser.
Remember that our Coco SSD model we used can only be a beginning point. If you want to continue exploring, the possibilities of the possibility of customizing object detection with TensorFlow.js that lets you tailor the app to identify the most appropriate objects for the requirements of your company.
There are endless possibilities! This app could serve as the foundation to develop more complex apps like Augmented Reality Experiences, as well as smart surveillance technologies. When you deploy your application through's secure platform, you are able to expose your creation to everyone around the globe and experience the capabilities of computer vision come into being.
What's a problem you've faced that you believe real-time object detection could solve? Share your thoughts in the comments below!
Kumar Harsh
Kumar is a designer of software and technical author who is located in India. He is a specialist in JavaScript in addition to DevOps. Get more details on his skills on his website.
This post was posted on here